Programming Languages: Principles and Paradigms
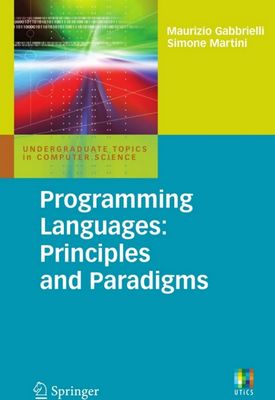
Lýsing:
This excellent addition to the UTiCS series of undergraduate textbooks provides a detailed and up to date description of the main principles behind the design and implementation of modern programming languages. Rather than focusing on a specific language, the book identifies the most important principles shared by large classes of languages. To complete this general approach, detailed descriptions of the main programming paradigms, namely imperative, object-oriented, functional and logic are given, analysed in depth and compared.
This provides the basis for a critical understanding of most of the programming languages. An historical viewpoint is also included, discussing the evolution of programming languages, and to provide a context for most of the constructs in use today. The book concludes with two chapters which introduce basic notions of syntax, semantics and computability, to provide a completely rounded picture of what constitutes a programming language.
Annað
- Höfundar: Maurizio Gabbrielli, Simone Martini
- Útgáfudagur: 2010-03-23
- Engar takmarkanir á útprentun
- Engar takmarkanir afritun
- Format:Page Fidelity
- ISBN 13: 9781848829145
- Print ISBN: 9781848829138
- ISBN 10: 1848829140
Efnisyfirlit
- Foreword
- Introduction
- Use of the text
- Acknowledgements
- Contents
- Abstract Machines
- The Concepts of Abstract Machine and of Interpreter
- The Interpreter
- An Example of an Abstract Machine: The Hardware Machine
- Memory
- The language of the physical machine
- Interpreter
- The Concepts of Abstract Machine and of Interpreter
- Implementation of a Language
- Implementation of an Abstract Machine
- Implementation in Hardware
- Simulation Using Software
- Emulation Using Firmware
- Implementation: The Ideal Case
- Notation
- Purely interpreted implementation
- Purely compiled implementation
- Comparing the Two Techniques
- Notation
- Implementation: The Real Case and The Intermediate Machine
- Implementation of an Abstract Machine
- Hierarchies of Abstract Machines
- Chapter Summary
- Bibliographic Notes
- Exercises
- References
- Levels of Description
- Grammar and Syntax
- Context-Free Grammars
- BNF
- Derivations and languages
- Derivation Trees
- Ambiguity
- Context-Free Grammars
- Lexical analysis
- Syntactic analysis
- Semantic analysis
- Generation of intermediate forms
- Code optimisation
- Code generation
- State
- Transitions
- Expression semantics
- Command semantics
- Computations
- The Halting Problem
- Expressiveness of Programming Languages
- Formalisms for Computability
- There are More Functions than Algorithms
- Chapter Summary
- Bibliographical Notes
- Exercises
- References
- Names and Denotable Objects
- Denotable Objects
- Environments and Blocks
- Blocks
- Types of Environment
- Operations on Environments
- Scope Rules
- Static Scope
- Dynamic Scope
- Some Scope Problems
- Chapter Summary
- Bibliographical Notes
- Exercises
- References
- Techniques for Memory Management
- Static Memory Management
- Dynamic Memory Management Using Stacks
- Activation Records for In-line Blocks
- Activation Records for Procedures
- Stack Management
- Dynamic Management Using a Heap
- Fixed-Length Blocks
- Variable-Length Blocks
- Single free list
- Multiple free lists
- Static Scope: The Static Chain
- Static Scope: The Display
- Dynamic Scope: Association Lists and CRT
- Central Referencing environment Table (CRT)
- Expressions
- Expression Syntax
- Infix Notation
- Prefix Notation
- Postfix Notation
- Semantics of Expressions
- Infix Notation: Precedence and Associativity
- Prefix Notation
- Postfix Notation
- Evaluation of Expressions
- Subexpression Evaluation Order
- Expression Syntax
- The Variable
- Assignment
- Commands for Explicit Sequence Control
- Sequential Command
- Composite Command
- Goto
- Other sequence control commands
- Conditional Commands
- If
- Case
- Iterative Commands
- Unbounded iteration
- Bounded iteration
- Expressiveness of bounded iteration
- The for in C
- For-each
- Tail Recursion
- Recursion or Iteration?
- Subprograms
- Parameters
- Return value
- Nonlocal environment
- Functional Abstraction
- Parameter Passing
- Call by value
- Call by reference
- Call by constant
- Call by result
- Call by value-result
- Call by name
- Functions as Parameters
- Implementation of deep binding
- Binding policy and static scope
- What defines the environment
- Functions as Results
- Pragmatics
- Implementing Exceptions
- Data Types
- Types as Support for Conceptual Organisation
- Types for Correctness
- Types and Implementation
- Type Systems
- Static and Dynamic Checking
- Scalar Types
- Booleans
- Characters
- Integers
- Reals
- Fixed Point
- Complex
- Void
- Enumerations
- Intervals
- Ordered Types
- Composite Types
- Records
- Variant Records and Unions
- Unions in C
- Variants and Security
- Arrays
- Operations on Arrays
- Checking
- Storage and Calculation of Indices
- Form of an Array: Where an Array is Allocated
- Dope Vectors
- Sets
- Pointers
- Pointer Arithmetic
- Deallocation
- Recursive Types
- Functions
- Equivalence
- Equivalence by Name
- Structural Equivalence
- Compatibility and Conversion
- Coercions
- Explicit Conversions
- Polymorphism
- Overloading
- Universal Parametric Polymorphism
- Explicit polymorphism
- Implicit Polymorphism
- Subtype Universal Polymorphism
- Remarks on the Implementation
- Type Checking and Inference
- Safety: An Evaluation
- Avoiding Dangling References
- Tombstone
- Locks and Keys
- Garbage Collection
- Reference Counting
- Mark and Sweep
- Interlude: Pointer Reversal
- Mark and Compact
- Copy
- Chapter Summary
- Bibliographic Notes
- Exercises
- References
- Abstract Data Types
- Information Hiding
- Representation Independence
- Modules
- Chapter Summary
- Bibliographical Notes
- Exercises
- References
- The Limits of Abstract Data Types
- A First Review
- Fundamental Concepts
- Objects
- Classes
- Objects in the heap and on the stack
- Encapsulation
- Subtypes
- Redefinition of a method
- Shadowing
- Abstract classes
- The subtype relation
- Constructors
- Inheritance
- Inheritance and visibility
- Single and multiple inheritance
- Dynamic Method Lookup
- Implementation Aspects
- Objects
- Classes and inheritance
- Late binding of self
- Single Inheritance
- Downcasting
- The Problem of Fragile Base Class
- Dynamic Method Dispatch in the JVM
- Multiple Inheritance
- Vtable Structure
- Replicated multiple inheritance
- Shared multiple inheritance
- Subtype Polymorphism
- Generics in Java
- Implementation of Generics in Java
- Generics, Arrays and Subtype Hierarchy
- Covariant and Contravariant Overriding
- Supertypes and Views
- Computations without State
- Expressions and Functions
- Computation as Reduction
- The Fundamental Ingredients
- Evaluation
- Values
- Capture-Free Substitution
- Evaluation Strategies
- Evaluation by value
- Evaluation by name
- Lazy evaluation
- Comparison of the Strategies
- Programming in a Functional Language
- Local Environment
- Interactiveness
- Types
- Pattern Matching
- Infinite Objects
- Imperative Aspects
- Implementation: The SECD Machine
- The Functional Paradigm: An Assessment
- Program Correctness
- Program schemata
- Assessment
- Fundamentals: The lambda-calculus
- Syntactic conventions, free and bound variables
- Substitution
- Alpha equivalence
- Computation: beta reduction
- Normal forms
- Confluence
- Fixpoint operators
- Expressiveness of the lambda-calculus
- Chapter Summary
- Bibliographical Note
- Exercises
- References
- Deduction as Computation
- Terminological Note
- An Example
- Syntax
- The Language of First-Order Logic
- Alphabet
- Terms
- Formulæ
- Logic Programs
- The Language of First-Order Logic
- Theory of Unification
- The Logic Variable
- Substitution
- Most General Unifier
- A Unification Algorithm
- Martelli and Montanari's unification algorithm
- The Herbrand Universe
- Declarative and Procedural Interpretation
- Procedure Calls
- Evaluation of a non-atomic goal
- Heads with arbitrary terms
- Control: Non-determinism
- Backtracking in Prolog
- Some Examples
- Prolog
- Arithmetic
- Cut
- Disjunction
- If-then-else
- Negation
- Logic Programming and Databases
- Logic Programming with Constraints
- Beginnings
- Factors in the Development of Languages
- 1950s and 60s
- FORTRAN
- Algol
- LISP
- COBOL
- Simula
- The 1970s
- C
- Pascal
- Smalltalk
- Declarative languages
- ML
- PROLOG
- The 1980s
- C++
- Ada
- CLP
- 1990s
- Java
- Chapter Summary
- Bibliographical Notes
- References
UM RAFBÆKUR Á HEIMKAUP.IS
Bókahillan þín er þitt svæði og þar eru bækurnar þínar geymdar. Þú kemst í bókahilluna þína hvar og hvenær sem er í tölvu eða snjalltæki. Einfalt og þægilegt!Rafbók til eignar
Rafbók til eignar þarf að hlaða niður á þau tæki sem þú vilt nota innan eins árs frá því bókin er keypt.
Þú kemst í bækurnar hvar sem er
Þú getur nálgast allar raf(skóla)bækurnar þínar á einu augabragði, hvar og hvenær sem er í bókahillunni þinni. Engin taska, enginn kyndill og ekkert vesen (hvað þá yfirvigt).
Auðvelt að fletta og leita
Þú getur flakkað milli síðna og kafla eins og þér hentar best og farið beint í ákveðna kafla úr efnisyfirlitinu. Í leitinni finnur þú orð, kafla eða síður í einum smelli.
Glósur og yfirstrikanir
Þú getur auðkennt textabrot með mismunandi litum og skrifað glósur að vild í rafbókina. Þú getur jafnvel séð glósur og yfirstrikanir hjá bekkjarsystkinum og kennara ef þeir leyfa það. Allt á einum stað.
Hvað viltu sjá? / Þú ræður hvernig síðan lítur út
Þú lagar síðuna að þínum þörfum. Stækkaðu eða minnkaðu myndir og texta með multi-level zoom til að sjá síðuna eins og þér hentar best í þínu námi.
Fleiri góðir kostir
- Þú getur prentað síður úr bókinni (innan þeirra marka sem útgefandinn setur)
- Möguleiki á tengingu við annað stafrænt og gagnvirkt efni, svo sem myndbönd eða spurningar úr efninu
- Auðvelt að afrita og líma efni/texta fyrir t.d. heimaverkefni eða ritgerðir
- Styður tækni sem hjálpar nemendum með sjón- eða heyrnarskerðingu
- Gerð : 208
- Höfundur : 9803
- Útgáfuár : 2010
- Leyfi : 380