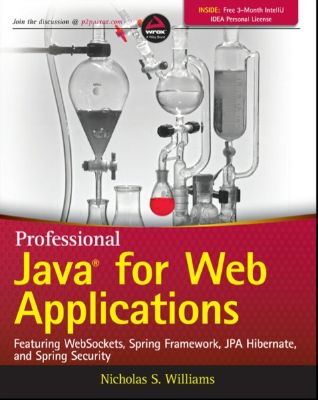
Lýsing:
Professional Java for Web Applications is a comprehensive title that developers can read cover-to-cover when building a complex Java application in an enterprise environment, or pick apart into sections when more knowledge about a particular topic is desired. Starting with an introduction to the Java Enterprise Edition and the basic web application, readers set up a development application server environment, learn about the tools needed during the development process, and explore numerous Java technologies and practices, including:* Application logging* JSR-303 bean validation* JSR-317 Java Persistence API (with Hibernate)* Spring Framework* Spring Security* Custom JSP tag libraries* WebSocket persistent, fully duplex web connections (new to Java EE 7)Each unit explores a new facet of enterprise application development that builds on the previous unit and enables the reader to jump to a particular point of interest or return to a unit in the future.
Readers will not only learn about the industry-standard tools and technologies used in enterprise development, but also enjoy an end-to-end guide to build an enterprise Java application from the ground up, and will explore both specific technologies and the programming concepts that they are built upon. The book is ideal for software developers familiar with Java who have been asked to build enterprise Java applications or would like to expand capabilities in Java to cover enterprise Java applications and web applications.
Annað
- Höfundur: Nicholas S. Williams
- Útgáfa:1
- Útgáfudagur: 2014-02-21
- Blaðsíður: 936
- Hægt að prenta út 2 bls.
- Hægt að afrita 10 bls.
- Format:Page Fidelity
- ISBN 13: 9781118656518
- Print ISBN: 9781118656464
- ISBN 10: 1118656512
Efnisyfirlit
- Professional: Java® for Web Applications
- Copyright
- About the Author
- About the Technical Editors
- Credits
- Acknowledgments
- Contents
- Introduction
- Who This Book Is For
- Who This Book Is Not For
- What You Will Learn in This Book
- Part I: Creating Enterprise Applications
- Part II: Adding Spring Framework Into the Mix
- Part III: Persisting Data with JPA and Hibernate ORM
- Part IV: Securing Your Application with Spring Security
- What You Will Not Learn in This Book
- What Tools You Will Need
- Java Development Kit for Java SE 8
- Integrated Development Environment
- NetBeans IDE 8.0
- Eclipse Luna IDE 4.4 for Java EE Developers
- IntelliJ IDEA 13 Ultimate Edition
- Java EE 7 Web Container
- Conventions Used in This Book
- Code Examples
- Maven Dependencies
- Why Security Is at the End of the Book
- Errata
- Part 1: Creating Enterprise Applications
- Chapter 1: Introducing Java Platform, Enterprise Edition
- A Timeline of Java Platforms
- In the Beginning
- The Birth of Enterprise Java
- Java SE and Java EE Evolving Together
- Understanding the Most Recent Platform Features
- A Continuing Evolution
- Understanding the Basic Web Application Structure
- Servlets, Filters, Listeners, and JSPs
- Directory Structure and WAR Files
- The Deployment Descriptor
- Class Loader Architecture
- Enterprise Archives
- Summary
- A Timeline of Java Platforms
- Chapter 2: Using Web Containers
- Choosing a Web Container
- Apache Tomcat
- GlassFish
- JBoss and WildFly
- Other Containers and Application Servers
- Why You'll Use Tomcat in This Book
- Installing Tomcat on Your Machine
- Installing as a Windows Service
- Installing as a Command-Line Application
- Configuring a Custom JSP Compiler
- Deploying and Undeploying Applications in Tomcat
- Performing a Manual Deploy and Undeploy
- Using the Tomcat Manager
- Debugging Tomcat from Your IDE
- Using IntelliJ IDEA
- Using Eclipse
- Summary
- Choosing a Web Container
- Chapter 3: Writing Your First Servlet
- Creating a Servlet Class
- What to Extend
- Using the Initializer and Destroyer
- Configuring a Servlet for Deployment
- Adding the Servlet to the Descriptor
- Mapping the Servlet to a URL
- Running and Debugging Your Servlet
- Understanding doGet(), doPost(), and Other Methods
- What Should Happen during the service Method Execution?
- Using HttpServletRequest
- Using HttpServletResponse
- Using Parameters and Accepting Form Submissions
- Configuring your Application Using Init Parameters
- Using Context Init Parameters
- Using Servlet Init Parameters
- Uploading Files from a Form
- Introducing the Customer Support Project
- Configuring the Servlet for File Uploads
- Accepting a File Upload
- Making Your Application Safe for Multithreading
- Understanding Requests, Threads, and Method Execution
- Protecting Shared Resources
- Summary
- Creating a Servlet Class
- Chapter:4 Using JSPs to Display Content
- <br /> Is Easier Than output.println("<br />")
- Why JSPs Are Better
- What Happens to a JSP at Run Time
- Creating Your First JSP
- Understanding the File Structure
- Directives, Declarations, Scriptlets, and Expressions
- Commenting Your Code
- Adding Imports to Your JSP
- Using Directives
- Using the <jsp> Tag
- Using Java within a JSP (and Why You Shouldn't!)
- Using the Implicit Variables in a JSP
- Why You Shouldn't Use Java in a JSP
- Combining Servlets and JSPs
- Configuring JSP Properties in the Deployment Descriptor
- Forwarding a Request from a Servlet to a JSP
- A Note about JSP Documents (JSPX)
- Summary
- <br /> Is Easier Than output.println("<br />")
- Chapter 5: Maintaining State Using Sessions
- Understanding Why Sessions Are Necessary
- Maintaining State
- Remembering Users
- Enabling Application Workflow
- Using Session Cookies and URL Rewriting
- Understanding the Session Cookie
- Session IDs in the URL
- Session Vulnerabilities
- Storing Data in a Session
- Configuring Sessions in the Deployment Descriptor
- Storing and Retrieving Data
- Removing Data
- Storing More Complex Data in Sessions
- Applying Sessions Usefully
- Adding Login to the Customer Support Application
- Detecting Changes to Sessions Using Listeners
- Maintaining a List of Active Sessions
- Clustering an Application That Uses Sessions
- Using Session IDs in a Cluster
- Understand Session Replication and Failover
- Summary
- Understanding Why Sessions Are Necessary
- Chapter 6: Using the Expression Language in JSPs
- Understanding Expression Language
- What It's For
- Understanding the Base Syntax
- Placing EL Expressions
- Writing with the EL Syntax
- Reserved Keywords
- Operator Precedence
- Object Properties and Methods
- EL Functions
- Static Field and Method Access
- Enums
- Lambda Expressions
- Collections
- Using Scoped Variables in EL Expressions
- Using the Implicit EL Scope
- Using the Implicit EL Variables
- Accessing Collections with the Stream API
- Understanding Intermediate Operations
- Using Terminal Operations
- Putting the Stream API to Use
- Replacing Java Code with Expression Language
- Summary
- Understanding Expression Language
- Chapter 7: Using the Java Standard Tag Library
- Introducing JSP Tags and the JSTL
- Working with Tags
- Using the Core Tag Library (C Namespace)
- <c:out>
- <c:url>
- <c:if>
- <c:choose>, <c:when>, and <c:otherwise>
- <c:forEach>
- <c:forTokens>
- <c:redirect>
- <c:import>
- <c:set> and <c:remove>
- Putting Core Library Tags to Use
- Using the Internationalization and Formatting Tag Library (FMT Namespace)
- Internationalization and Localization Components
- <fmt:message>
- <fmt:setLocale>
- <fmt:bundle> and <fmt:setBundle>
- <fmt:requestEncoding>
- <fmt:timeZone> and <fmt:setTimeZone>
- <fmt:formatDate> and <fmt:parseDate>
- <fmt:formatNumber> and <fmt:parseNumber>
- Putting i18n and Formatting Library Tags to Use
- Using the Database Access Tag Library (SQL Namespace)
- Using the XML Processing Tag Library (X Namespace)
- Replacing Java Code with JSP Tags
- Summary
- Introducing JSP Tags and the JSTL
- Chapter 8: Writing Custom Tag and Function Libraries
- Understanding TLDs, Tag Files, and Tag Handlers
- Reading the Java Standard Tag Library TLD
- Comparing JSP Directives and Tag File Directives
- Creating Your First Tag File to Serve as an HTML Template
- Creating a More Useful Date Formatting Tag Handler
- Creating an EL Function to Abbreviate Strings
- Replacing Java Code with Custom JSP Tags
- Summary
- Understanding TLDs, Tag Files, and Tag Handlers
- Chapter 9: Improving Your Application Using Filters
- Understanding the Purpose of Filters
- Logging Filters
- Authentication Filters
- Compression and Encryption Filters
- Error Handling Filters
- Creating, Declaring, and Mapping Filters
- Understanding the Filter Chain
- Mapping to URL Patterns and Servlet Names
- Mapping to Different Request Dispatcher Types
- Using the Deployment Descriptor
- Using Annotations
- Using Programmatic Configuration
- Ordering Your Filters Properly
- URL Pattern Mapping versus Servlet Name Mapping
- Exploring Filter Order with a Simple Example
- Using Filters with Asynchronous Request Handling
- Investigating Practical Uses for Filters
- Adding a Simple Logging Filter
- Compressing Response Content Using a Filter
- Simplifying Authentication with a Filter
- Summary
- Understanding the Purpose of Filters
- Chapter 10: Making Your Application Interactive with WebSockets
- Evolution: From Ajax to WebSockets
- Problem: Getting New Data from the Server to the Browser
- Solution 1: Frequent Polling
- Solution 2: Long Polling
- Solution 3: Chunked Encoding
- Solution 4: Applets and Adobe Flash
- WebSockets: The Solution Nobody Knew Kind of Already Existed
- Understanding the WebSocket APIS
- HTML5 (JavaScript) Client API
- Java WebSocket APIs
- Creating Multiplayer Games with WebSockets
- Implementing the Basic Tic-Tac-Toe Algorithm
- Creating the Server Endpoint
- Writing the JavaScript Game Console
- Playing WebSocket Tic-Tac-Toe
- Using WebSockets to Communicate in a Cluster
- Simulating a Simple Cluster Using Two Servlet Instances
- Transmitting and Receiving Binary Messages
- Testing the Simulated Cluster Application
- Adding "Chat with Support" to the Customer Support Application
- Using Encoders and Decoders to Translate Messages
- Creating the Chat Server Endpoint
- Writing the JavaScript Chat Application
- Summary
- Evolution: From Ajax to WebSockets
- Chapter 11: Using Logging to Monitor Your Application
- Understanding the Concepts of Logging
- Why You Should Log
- What Content You Might Want to See in Logs
- How Logs Are Written
- Using Logging Levels and Categories
- Why Are There Different Logging Levels?
- Logging Levels Defined
- How Logging Categories Work
- How Log Sifting Works
- Choosing a Logging Framework
- API versus Implementation
- Performance
- A Quick Look at Apache Commons Logging and SLF4J
- Introducing Log4j 2
- Integrating Logging into Your Application
- Creating the Log4j 2 Configuration Files
- Utilizing Fish Tagging with a Web Filter
- Writing Logging Statements in Java Code
- Using the Log Tag Library in JSPs
- Logging in the Customer Support Application
- Summary
- Understanding the Concepts of Logging
- Chapter 1: Introducing Java Platform, Enterprise Edition
- Chapter 12: Introducing Spring Framework
- What Is Spring Framework?
- Inversion of Control and Dependency Injection
- Aspect-Oriented Programming
- Data Access and Transaction Management
- Application Messaging
- Model-View-Controller Pattern for Web Applications
- Why Spring Framework?
- Logical Code Groupings
- Multiple User Interfaces Utilizing One Code Base
- Understanding Application Contexts
- Bootstrapping Spring Framework
- Using the Deployment Descriptor to Bootstrap Spring
- Programmatically Bootstrapping Spring in an Initializer
- Configuring Spring Framework
- Creating an XML Configuration
- Creating a Hybrid Configuration
- Configuring Spring with Java Using @Configuration
- Utilizing Bean Definition Profiles
- Understanding How Profiles Work
- Considering Antipatterns and Security Concerns
- Summary
- What Is Spring Framework?
- Chapter 13: Replacing Your Servlets with Controllers
- Understanding @RequestMapping
- Using @RequestMapping Attributes to Narrow Request Matching
- Specifying Controller Method Parameters
- Selecting Valid Return Types for Controller Methods
- Using Spring Framework's Model and View Pattern
- Using Explicit Views and View Names
- Using Implicit Views with Model Attributes
- Returning Response Entities
- Making Your Life Easier with Form Objects
- Adding the Form Object to Your Model
- Using the Spring Framework <form> Tags
- Obtaining Submitted Form Data
- Updating the Customer Support Application
- Enabling Multipart Support
- Converting Servlets to Spring MVC Controllers
- Creating a Custom Downloading View
- Summary
- Understanding @RequestMapping
- Chapter 14: Using Services and Repositories to Support Your Controllers
- Understanding Model-View-Controller Plus Controller-Service-Repository
- Recognizing Different Types of Program Logic
- Repositories Provide Persistence Logic
- Services Provide Business Logic
- Controllers Provide User Interface Logic
- Using the Root Application Context Instead of a Web Application Context
- Reusing the Root Application Context for Multiple User Interfaces
- Moving Your Business Logic from Controllers to Services
- Using Repositories for Data Storage
- Improving Services with Asynchronous and Scheduled Execution
- Understanding Executors and Schedulers
- Configuring a Scheduler and Asynchronous Support
- Creating and Using @Async Methods
- Creating and Using @Scheduled Methods
- Applying Logic Layer Separation to WebSockets
- Adding Container-Managed Objects to the Spring Application Context
- Using the Spring WebSocket Configurator
- Remember: A WebSocket Is Just Another Interface for Business Logic
- Summary
- Understanding Model-View-Controller Plus Controller-Service-Repository
- Chapter 15: Internationalizing Your Application with Spring Framework i18n
- Why Do You Need Spring Framework i18n?
- Making Internationalization Easier
- Localizing Error Messages Directly
- Using the Basic Internationalization and Localization APIS
- Understanding Resource Bundles and Message Formats
- Message Sources to the Rescue
- Using Message Sources to Internationalize JSPs
- Con"guring Internationalization in Spring Framework
- Creating a Message Source
- Understanding Locale Resolvers
- Using a Handler Interceptor to Change Locales
- Providing a User Profile Locale Setting
- Including Time Zone Support
- Understanding How Themes Can Improve Internationalization
- Internationalizing Your Code
- Using the <spring:message> Tag
- Handling Application Errors Cleanly
- Updating the Customer Support Application
- Using the Message Source Directly
- Summary
- Why Do You Need Spring Framework i18n?
- Chapter 16: Using JSR 349, Spring Framework, and Hibernate Validator for Bean Validation
- What Is Bean Validation?
- Why Hibernate Validator?
- Understanding the Annotation Metadata Model
- Using Bean Validation with Spring Framework
- Configuring Validation in the Spring Framework Container
- Configuring the Spring Validator Bean
- Setting Up Error Code Localization
- Using a Method Validation Bean Post-Processor
- Making Spring MVC Use the Same Validation Beans
- Adding Constraint Validation Annotations to Your Beans
- Understanding the Built-in Constraint Annotations
- Understanding Common Constraint Attributes
- Putting Constraints to Use
- Using @Valid for Recursive Validation
- Using Validation Groups
- Checking Constraint Legality at Compile-Time
- Configuring Spring Beans for Method Validation
- Annotating Interfaces, Not Implementations
- Using Constraints and Recursive Validation on Method Parameters
- Validating Method Return Values
- Indicating That a Class Is Eligible for Method Validation
- Using Parameter Validation in Spring MVC Controllers
- Displaying Validation Errors to the User
- Writing Your Own Validation Constraints
- Inheriting Other Constraints in a Custom Constraint
- Creating a Constraint Validator
- Understanding the Constraint Validator Life Cycle
- Integrating Validation in the Customer Support Application
- Summary
- What Is Bean Validation?
- Chapter 17: Creating RESTful and SOAP Web Services
- Understanding Web Services
- In the Beginning There Was SOAP
- RESTful Web Services Provide a Simpler Approach
- Con"guring RESTful Web Services with Spring MVC
- Segregating Controllers with Stereotype Annotations
- Creating Separate Web and REST Application Contexts
- Handling Error Conditions in RESTful Web Services
- Mapping RESTful Requests to Controller Methods
- Improving Discovery with an Index Endpoint
- Testing Your Web Service Endpoints
- Choosing a Testing Tool
- Making Requests to Your Web Service
- Using Spring Web Services for SOAP
- Writing Your Contract-First XSD and WSDL
- Adding the SOAP Dispatcher Servlet Configuration
- Creating a SOAP Endpoint
- Summary
- Understanding Web Services
- Chapter 18: Using Messaging and Clustering for Flexibility and Reliability
- Recognizing When You Need Messaging and Clustering
- What Is Application Messaging?
- What Is Clustering?
- How Do Messaging and Clustering Work Together?
- Adding Messaging Support to your Application
- Creating Application Events
- Subscribing to Application Events
- Publishing Application Events
- Making your Messaging Distributable Across a Cluster
- Updating Your Events to Support Distribution
- Creating and Configuring a Custom Event Multicaster
- Using WebSockets to Send and Receive Events
- Discovering Nodes with Multicast Packets
- Simulating a Cluster with Multiple Deployments
- Distributing Events with AMQP
- Configuring an AMQP Broker
- Creating an AMQP Multicaster
- Running the AMQP-Enabled Application
- Summary
- Recognizing When You Need Messaging and Clustering
- Chapter 19: Introducing Java Persistence API and Hibernate ORM
- What Is Data Persistence?
- Flat-File Entity Storage
- Structured File Storage
- Relational Database Systems
- Object-Oriented Databases
- Schema-less Database Systems
- What Is an Object-Relational Mapper?
- Understanding the Problem of Persisting Entities
- O/RMs Make Entity Persistence Easier
- JPA Provides a Standard O/RM API
- Why Hibernate ORM?
- A Brief Look at Hibernate ORM
- Using Hibernate Mapping Files
- Understanding the Session API
- Getting a Session from the SessionFactory
- Creating a SessionFactory with Spring Framework
- Preparing a Relational Database
- Installing MySQL and MySQL Workbench
- Installing the MySQL JDBC Driver
- Creating a Connection Resource in Tomcat
- A Note About Maven Dependencies
- Summary
- What Is Data Persistence?
- Chapter 20: Mapping Entities to Tables with JPA Annotations
- Getting Started with Simple Entities
- Marking an Entity and Mapping It to a Table
- Indicating How JPA Uses Entity Fields
- Mapping Surrogate Keys
- Using Basic Data Types
- Specifying Column Names and Other Details
- Creating and Using a Persistence Unit
- Designing the Database Tables
- Understanding Persistence Unit Scope
- Creating the Persistence Configuration
- Using the Persistence API
- Mapping Complex Data Types
- Using Enums as Entity Properties
- Understanding How JPA Handles Dates and Times
- Mapping Large Properties to CLOBs and BLOBs
- Summary
- Getting Started with Simple Entities
- Chapter 21: Using JPA in Spring Framework Repositories
- Using Spring Repositories and Transactions
- Understanding Transaction Scope
- Using Threads for Transactions and Entity Managers
- Taking Advantage of Exception Translation
- Configuring Persistence in Spring Framework
- Looking Up a Data Source
- Creating a Persistence Unit in Code
- Setting Up Transaction Management
- Creating and Using JPA Repositories
- Injecting the Persistence Unit
- Implementing Standard CRUD Operations
- Creating a Base Repository for All Your Entities
- Demarking Transaction Boundaries in Your Services
- Using the Transactional Service Methods
- Converting Data with DTOs and Entities
- Creating Entities for the Customer Support Application
- Securing User Passwords with BCrypt
- Transferring Data to Entities in Your Services
- Summary
- Using Spring Repositories and Transactions
- Chapter 22: Eliminating Boilerplate Repositories with Spring Data JPA
- Understanding Spring Data's Uni"ed Data Access
- Avoiding Duplication of Code
- Using the Stock Repository Interfaces
- Creating Query Methods for Finding Entities
- Providing Custom Method Implementations
- Con"guring and Creating Spring Data JPA Repositories
- Enabling Repository Auto-Generation
- Writing and Using Spring Data JPA Interfaces
- Refactoring the Customer Support Application
- Converting the Existing Repositories
- Adding Comments to Support Tickets
- Summary
- Understanding Spring Data's Uni"ed Data Access
- Chapter 23: Searching for Data with JPA and Hibernate Search
- An Introduction to Searching
- Understanding the Importance of Indexes
- Taking Three Different Approaches
- Using Advanced Criteria to Locate Objects
- Creating Complex Criteria Queries
- Using OR in Your Queries
- Creating Useful Indexes to Improve Performance
- Taking Advantage of Full-Text Indexes with JPA
- Creating Full-Text Indexes in MySQL Tables
- Creating and Using a Searchable Repository
- Making Full-Text Searching Portable
- Indexing Any Data with Apache Lucene and Hibernate Search
- Understanding Lucene Full-Text Indexing
- Annotating Entities with Indexing Metadata
- Using Hibernate Search with JPA
- Summary
- An Introduction to Searching
- Chapter 24: Creating Advanced Mappings and Custom Data Types
- What's Left?
- Converting Nonstandard Data Types
- Understanding Attribute Converters
- Understanding the Conversion Annotations
- Creating and Using Attribute Converters
- Embedding POJOs Within Entities
- Indicating That a Type Is Embeddable
- Marking a Property as Embedded
- Overriding Embeddable Column Names
- Defining Relationships Between Entities
- Understanding One-to-One Relationships
- Using One-to-Many and Many-to-One Relationships
- Creating Many-to-Many Relationships
- Addressing Other Common Situations
- Versioning Entities with Revisions and Timestamps
- Defining Abstract Entities with Common Properties
- Mapping Basic and Embedded Collections
- Persisting a Map of Key-Value Pairs
- Storing an Entity in Multiple Tables
- Creating Programmatic Triggers
- Acting before and after CRUD Operations
- Using Entity Listeners
- Refining the Customer Support Application
- Mapping a Collection of Attachments
- Lazy Loading Simple Properties with Load Time Weaving
- Summary
- Chapter 25: Introducing Spring Security
- What Is Authentication?
- Integrating Authentication
- Understanding Authorization
- Why Spring Security?
- Understanding the Spring Security Foundation
- Using Spring Security's Authorization Services
- Configuring Spring Security
- Summary
- What Is Authentication?
- Chapter 26: Authenticating Users with Spring Security
- Choosing and Configuring an Authentication Provider
- Configuring a User Details Provider
- Working with LDAP and Active Directory Providers
- Authenticating with OpenID
- Remembering Users
- Exploring Other Authentication Providers
- Writing Your Own Authentication Provider
- Bootstrapping in the Correct Order
- Creating and Configuring a Provider
- Mitigating Cross-Site Request Forgery Attacks
- Summary
- Choosing and Configuring an Authentication Provider
- Chapter 27: Using Authorization Tags and Annotations
- Authorizing by Declaration
- Checking Permissions in Method Code
- Employing URL Security
- Using Annotations to Declare Permissions
- Defining Method Pointcut Rules
- Understanding Authorization Decisions
- Using Access Decision Voters
- Using Access Decision Managers
- Creating Access Control Lists for Object Security
- Understanding Spring Security ACLs
- Configuring Access Control Lists
- Populating ACLs for Your Entities
- Adding Authorization to Customer Support
- Switching to Custom User Details
- Securing Your Service Methods
- Using Spring Security's Tag Library
- Summary
- Authorizing by Declaration
- Chapter 28: Securing RESTful Web Services with OAuth
- Understanding Web Service Security
- Comparing Web GUI and Web Service Security
- Choosing an Authentication Mechanism
- Introducing OAuth
- Understanding the Key Players
- The Beginning: OAuth 1.0
- The Standard: OAuth 1.0a
- The Evolution: OAuth 2.0
- Using Spring Security OAuth
- Creating on OAuth 2.0 Provider
- Creating an OAuth 2.0 Client
- Finishing the Customer Support Application
- Generating Request Nonces and Signatures
- Implementing Client Services
- Implementing Nonce Services
- Implementing Token Services
- Customizing the Resource Server Filter
- Reconfiguring Spring Security
- Creating an OAuth Client Application
- Customizing the REST Template
- Configuring the Spring Security OAuth Client
- Using the REST Template
- Testing the Provider and Client Together
- Summary
- Understanding Web Service Security
UM RAFBÆKUR Á HEIMKAUP.IS
Bókahillan þín er þitt svæði og þar eru bækurnar þínar geymdar. Þú kemst í bókahilluna þína hvar og hvenær sem er í tölvu eða snjalltæki. Einfalt og þægilegt!Rafbók til eignar
Rafbók til eignar þarf að hlaða niður á þau tæki sem þú vilt nota innan eins árs frá því bókin er keypt.
Þú kemst í bækurnar hvar sem er
Þú getur nálgast allar raf(skóla)bækurnar þínar á einu augabragði, hvar og hvenær sem er í bókahillunni þinni. Engin taska, enginn kyndill og ekkert vesen (hvað þá yfirvigt).
Auðvelt að fletta og leita
Þú getur flakkað milli síðna og kafla eins og þér hentar best og farið beint í ákveðna kafla úr efnisyfirlitinu. Í leitinni finnur þú orð, kafla eða síður í einum smelli.
Glósur og yfirstrikanir
Þú getur auðkennt textabrot með mismunandi litum og skrifað glósur að vild í rafbókina. Þú getur jafnvel séð glósur og yfirstrikanir hjá bekkjarsystkinum og kennara ef þeir leyfa það. Allt á einum stað.
Hvað viltu sjá? / Þú ræður hvernig síðan lítur út
Þú lagar síðuna að þínum þörfum. Stækkaðu eða minnkaðu myndir og texta með multi-level zoom til að sjá síðuna eins og þér hentar best í þínu námi.
Fleiri góðir kostir
- Þú getur prentað síður úr bókinni (innan þeirra marka sem útgefandinn setur)
- Möguleiki á tengingu við annað stafrænt og gagnvirkt efni, svo sem myndbönd eða spurningar úr efninu
- Auðvelt að afrita og líma efni/texta fyrir t.d. heimaverkefni eða ritgerðir
- Styður tækni sem hjálpar nemendum með sjón- eða heyrnarskerðingu
- Gerð : 208
- Höfundur : 12184
- Útgáfuár : 2014
- Leyfi : 379