Java: An Introduction to Problem Solving and Programming, Global Edition
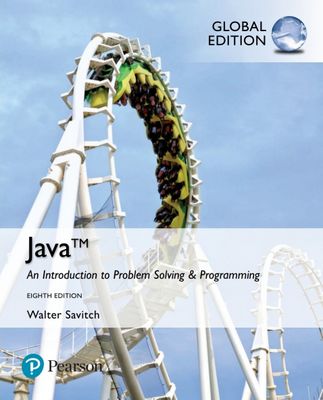
Lýsing:
For courses in introductory Computer Science courses using Java, and other introductory programming courses in Computer Science, Computer Engineering, CIS, MIS, IT, and Business. Ideal for a wide range of introductory computer science courses, Java: An Introduction to Problem Solving and Programming, 8th Edition introduces students to object-oriented programming and important concepts such as design, testing and debugging, programming style, interfaces and inheritance, and exception handling.
A concise, accessible introduction to Java, the text covers key Java language features in a manner that resonates with introductory programmers. Objects are covered early and thoroughly in the text. The author’s tried-and-true pedagogy incorporates numerous case studies, programming examples, and programming tips, while flexibility charts and optional graphics sections allow instructors to order chapters and sections based on their course needs.
This 8th Edition incorporates new examples, updated material, and revisions. The full text downloaded to your computer With eBooks you can: search for key concepts, words and phrases make highlights and notes as you study share your notes with friends eBooks are downloaded to your computer and accessible either offline through the Bookshelf (available as a free download), available online and also via the iPad and Android apps.
Annað
- Höfundur: Walter Savitch
- Útgáfa:8
- Útgáfudagur: 2018-08-07
- Hægt að prenta út 2 bls.
- Hægt að afrita 2 bls.
- Format:Page Fidelity
- ISBN 13: 9781292247533
- Print ISBN: 9781292247472
- ISBN 10: 1292247533
Efnisyfirlit
- Inside Front Cover
- Title Page
- Copyright Page
- Preface for Instructors
- Preface for Students
- Acknowledgments
- Acknowledgments for the Global Edition
- Dependency Chart
- Brief Contents
- Contents
- Chapter 1 Introduction to Computers and Java
- 1.1 COMPUTER BASICS
- Hardware and Memory
- Programs
- Programming Languages, Compilers, and Interpreters
- Java Bytecode
- Class Loader
- 1.2 A SIP OF JAVA
- History of the Java Language
- Applications and Applets
- A First Java Application Program
- Writing, Compiling, and Running a Java Program
- 1.3 PROGRAMMING BASICS
- Object-Oriented Programming
- Algorithms
- Testing and Debugging
- Software Reuse
- 1.4 GRAPHICS SUPPLEMENT
- A Sample JavaFX Application
- Size and Position of Figures
- Drawing Ovals and Circles
- Drawing Arcs
- 1.1 COMPUTER BASICS
- 2.1 VARIABLES AND EXPRESSIONS
- Variables
- Data Types
- Java Identifiers
- Assignment Statements
- Simple Input
- Simple Screen Output
- Constants
- Named Constants
- Assignment Compatibilities
- Type Casting
- Arithmetic Operators
- Parentheses and Precedence Rules
- Specialized Assignment Operators
- Case Study: Vending Machine Change
- Increment and Decrement Operators
- More About the Increment and Decrement Operators
- 2.2 THE CLASS String
- String Constants and Variables
- Concatenation of Strings
- String Methods
- String Processing
- Escape Characters
- The Unicode Character Set
- 2.3 KEYBOARD AND SCREEN I/O
- Screen Output
- Keyboard Input
- Other Input Delimiters (Optional)
- Formatted Output with printf (Optional)
- 2.4 DOCUMENTATION AND STYLE
- Meaningful Variable Names
- Comments
- Indentation
- Using Named Constants
- 2.5 GRAPHICS SUPPLEMENT
- Style Rules Applied to a JavaFX Application
- Introducing the Class JOptionPane
- Reading Input as Other Numeric Types
- Programming Example: Change-Making Program with Windowing I/O
- 3.1 THE if-else STATEMENT
- The Basic if-else Statement
- Boolean Expressions
- Comparing Strings
- Nested if-else Statements
- Multibranch if-else Statements
- Programming Example: Assigning Letter Grades
- Case Study: Body Mass Index
- The Conditional Operator (Optional)
- The exit Method
- 3.2 THE TYPE boolean
- Boolean Variables
- Precedence Rules
- Input and Output of Boolean Values
- 3.3 THE switch STATEMENT
- Enumerations
- 3.4 GRAPHICS SUPPLEMENT
- Specifying a Drawing Color
- A Dialog Box for a Yes-or-No Question
- 4.1 JAVA LOOP STATEMENTS
- The while Statement
- The do-while Statement
- Programming Example: Bug Infestation
- Programming Example: Nested Loops
- The for Statement
- Declaring Variables Within a for Statement
- Using a Comma in a for Statement (Optional)
- The for-each Statement
- 4.2 PROGRAMMING WITH LOOPS
- The Loop Body
- Initializing Statements
- Controlling the Number of Loop Iterations
- Case Study: Using a Boolean Variable to End a Loop
- Programming Example: Spending Spree
- The break Statement and continue Statement in Loops (Optional)
- Loop Bugs
- Tracing Variables
- Assertion Checks
- 4.3 GRAPHICS SUPPLEMENT
- Programming Example: A Multiface JavaFX Application
- Drawing Text
- 5.1 CLASS AND METHOD DEFINITIONS
- Class Files and Separate Compilation
- Programming Example: Implementing a Dog Class
- Instance Variables
- Methods
- Defining void Methods
- Defining Methods That Return a Value
- Programming Example: First Try at Implementing a Species Class
- The Keyword this
- Local Variables
- Blocks
- Parameters of a Primitive Type
- Class Files and Separate Compilation
- 5.2 INFORMATION HIDING AND ENCAPSULATION
- Information Hiding
- Precondition and Postcondition Comments
- The public and private Modifiers
- Programming Example: A Demonstration of Why Instance Variables Should Be Private
- Programming Example: Another Implementation of a Class of Rectangles
- Accessor Methods and Mutator Methods
- Programming Example: A Purchase Class
- Methods Calling Methods
- Encapsulation
- Automatic Documentation with javadoc
- UML Class Diagrams
- 5.3 OBJECTS AND REFERENCES
- Variables of a Class Type
- Defining an equals Method for a Class
- Programming Example: A Species Class
- Boolean-Valued Methods
- Case Study: Unit Testing
- Parameters of a Class Type
- Programming Example: Class-Type Parameters Versus Primitive-Type Parameters
- The GraphicsContext Class
- Programming Example: Multiple Faces, but with a Helping Method
- Adding Labels to a JavaFX Application
- 6.1 CONSTRUCTORS
- Defining Constructors
- Calling Methods from Constructors
- Calling a Constructor from Other Constructors (Optional)
- 6.2 STATIC VARIABLES AND STATIC METHODS
- Static Variables
- Static Methods
- Dividing the Task of a main Method into Subtasks
- Adding a main Method to a Class
- The Math Class
- Wrapper Classes
- 6.3 WRITING METHODS
- Case Study: Formatting Output
- Decomposition
- Addressing Compiler Concerns
- Testing Methods
- 6.4 OVERLOADING
- Overloading Basics
- Overloading and Automatic Type Conversion
- Overloading and the Return Type
- Programming Example: A Class for Money
- Privacy Leaks
- Packages and Importing
- Package Names and Directories
- Name Clashes
- Adding Buttons
- Adding Icons
- 7.1 ARRAY BASICS
- Creating and Accessing Arrays
- Array Details
- The Instance Variable length
- More About Array Indices
- Initializing Arrays
- 7.2 ARRAYS IN CLASSES AND METHODS
- Case Study: Sales Report
- Indexed Variables as Method Arguments
- Entire Arrays as Arguments to a Method
- Arguments for the Method main
- Array Assignment and Equality
- Methods That Return Arrays
- 7.3 PROGRAMMING WITH ARRAYS AND CLASSES
- Programming Example: A Specialized List Class
- Partially Filled Arrays
- 7.4 SORTING AND SEARCHING ARRAYS
- Selection Sort
- Other Sorting Algorithms
- Searching an Array
- 7.5 MULTIDIMENSIONAL ARRAYS
- Multidimensional-Array Basics
- Multidimensional-Array Parameters and Returned Values
- Java’s Representation of Multidimensional Arrays
- Ragged Arrays (Optional)
- Programming Example: Employee Time Records
- Layout Panes
- Text Areas, Text Fields and Combining Layouts
- Drawing Polygons
- 8.1 INHERITANCE BASICS
- Derived Classes
- Overriding Method Definitions
- Overriding Versus Overloading
- The final Modifier
- Private Instance Variables and Private Methods of a Base Class
- UML Inheritance Diagrams
- 8.2 PROGRAMMING WITH INHERITANCE
- Constructors in Derived Classes
- The this Method—Again
- Calling an Overridden Method
- Programming Example: A Derived Class of a Derived Class
- Another Way to Define the equals Method in Undergraduate
- Type Compatibility
- The Class Object
- A Better equals Method
- 8.3 POLYMORPHISM
- Dynamic Binding and Inheritance
- Dynamic Binding with toString
- 8.4 INTERFACES AND ABSTRACT CLASSES
- Class Interfaces
- Java Interfaces
- Implementing an Interface
- An Interface as a Type
- Extending an Interface
- Case Study: Character Graphics
- Case Study: The Comparable Interface
- Abstract Classes
- 8.5 GRAPHICS SUPPLEMENT
- Event-Driven Programming
- Event Handling in a Separate Class
- Event Handling in the Main GUI Application Class
- Event Handling in an Anonymous Inner Class
- Programming Example: Adding Numbers
- 9.1 BASIC EXCEPTION HANDLING
- Exceptions in Java
- Predefined Exception Classes
- 9.2 DEFINING YOUR OWN EXCEPTION CLASSES
- 9.3 MORE ABOUT EXCEPTION CLASSES
- Declaring Exceptions (Passing the Buck)
- Kinds of Exceptions
- Errors
- Multiple Throws and Catches
- The finally Block
- Rethrowing an Exception (Optional)
- Case Study: A Line-Oriented Calculator
- Additional User Interface Controls and Shapes
- Images and Shapes
- Handling Mouse Events
- The Timeline Class
- 10.1 AN OVERVIEW OF STREAMS AND FILE I/O
- The Concept of a Stream
- Why Use Files for I/O?
- Text Files and Binary Files
- 10.2 TEXT-FILE I/O
- Creating a Text File
- Appending to a Text File
- Reading from a Text File
- 10.3 TECHNIQUES FOR ANY FILE
- The Class File
- Programming Example: Reading a File Name from the Keyboard
- Using Path Names
- Methods of the Class File
- Defining a Method to Open a Stream
- Case Study: Processing a Comma-Separated Values File
- The Class File
- Creating a Binary File
- Writing Primitive Values to a Binary File
- Writing Strings to a Binary File
- Some Details About writeUTF
- Reading from a Binary File
- The Class EOFException
- Programming Example: Processing a File of Binary Data
- Binary-File I/O with Objects of a Class
- Some Details of Serialization
- Array Objects in Binary Files
- Programming Example: A JavaFX GUI for Manipulating Files
- 11.1 THE BASICS OF RECURSION
- Case Study: Digits to Words
- How Recursion Works
- Infinite Recursion
- Recursive Methods Versus Iterative Methods
- Recursive Methods That Return a Value
- 11.2 PROGRAMMING WITH RECURSION
- Programming Example: Insisting That User Input Be Correct
- Case Study: Binary Search
- Programming Example: Merge Sort—A Recursive Sorting Method
- 11.3 GRAPHICS SUPPLEMENT
- Lambda Functions and Event Handlers
- 12.1 ARRAY-BASED DATA STRUCTURES
- The Class ArrayList
- Creating an Instance of ArrayList
- Using the Methods of ArrayList
- Programming Example: A To-Do List
- Parameterized Classes and Generic Data Types
- 12.2 THE JAVA COLLECTIONS FRAMEWORK
- The Collection Interface
- The Class HashSet
- The Map Interface
- The Class HashMap
- 12.3 LINKED DATA STRUCTURES
- The Class LinkedList
- Linked Lists
- Implementing the Operations of a Linked List
- A Privacy Leak
- Inner Classes
- Node Inner Classes
- Iterators
- The Java Iterator Interface
- Exception Handling with Linked Lists
- Variations on a Linked List
- Other Linked Data Structures
- 12.4 GENERICS
- The Basics
- Programming Example: A Generic Linked List
- The Basics
- Building JavaFX Applications with the Scene Builder
- Where to Go from Here
- 1 Getting Java
- 2 Running Applets
- 3 Protected and Package Modifiers
- 4 The DecimalFormat Class
- Other Pattern Symbols
- 5 Javadoc
- Commenting Classes for Use with javadoc
- Running javadoc
- 6 Differences Between C++ and Java
- Primitive Types
- Strings
- Flow of Control
- Testing for Equality
- main Method (Function) and Other Methods
- Files and Including Files
- Class and Method (Function) Definitions
- No Pointer Types in Java
- Method (Function) Parameters
- Arrays
- Garbage Collection
- Other Comparisons
- 7 Unicode Character Codes
- 8 Introduction to Java 8 Functional Programming
- 9 The Iterator Interface
- 10 Cloning
- 11 Java Reserved Keywords
- SYMBOLS
- A
- B
- C
- D
- E
- F
- G
- H
- I
- J
- K
- L
- M
- N
- O
- P
- Q
- R
- S
- T
- U
- V
- W
- X
- Y
- Z
UM RAFBÆKUR Á HEIMKAUP.IS
Bókahillan þín er þitt svæði og þar eru bækurnar þínar geymdar. Þú kemst í bókahilluna þína hvar og hvenær sem er í tölvu eða snjalltæki. Einfalt og þægilegt!Rafbók til eignar
Rafbók til eignar þarf að hlaða niður á þau tæki sem þú vilt nota innan eins árs frá því bókin er keypt.
Þú kemst í bækurnar hvar sem er
Þú getur nálgast allar raf(skóla)bækurnar þínar á einu augabragði, hvar og hvenær sem er í bókahillunni þinni. Engin taska, enginn kyndill og ekkert vesen (hvað þá yfirvigt).
Auðvelt að fletta og leita
Þú getur flakkað milli síðna og kafla eins og þér hentar best og farið beint í ákveðna kafla úr efnisyfirlitinu. Í leitinni finnur þú orð, kafla eða síður í einum smelli.
Glósur og yfirstrikanir
Þú getur auðkennt textabrot með mismunandi litum og skrifað glósur að vild í rafbókina. Þú getur jafnvel séð glósur og yfirstrikanir hjá bekkjarsystkinum og kennara ef þeir leyfa það. Allt á einum stað.
Hvað viltu sjá? / Þú ræður hvernig síðan lítur út
Þú lagar síðuna að þínum þörfum. Stækkaðu eða minnkaðu myndir og texta með multi-level zoom til að sjá síðuna eins og þér hentar best í þínu námi.
Fleiri góðir kostir
- Þú getur prentað síður úr bókinni (innan þeirra marka sem útgefandinn setur)
- Möguleiki á tengingu við annað stafrænt og gagnvirkt efni, svo sem myndbönd eða spurningar úr efninu
- Auðvelt að afrita og líma efni/texta fyrir t.d. heimaverkefni eða ritgerðir
- Styður tækni sem hjálpar nemendum með sjón- eða heyrnarskerðingu
- Gerð : 208
- Höfundur : 5724
- Útgáfuár : 2018
- Leyfi : 380